일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 |
- 롤 #리그오브레전드
- 깃허브 #깃 #커밋
- 라이엇 #api #롤api
- js #예약어 #자바스크랩트
- js #web
- js #제이쿼리 #비동기통신 #코딩 #프로그래밍
- 사이드프로젝트 #토이프로젝트 #개발 #개발자
- 코드업 #코딩문제
- ci #개발 #git
- Today
- Total
목록분류 전체보기 (39)
Just Do it
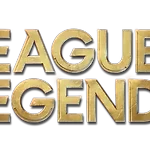
일단 여기로 들어간다 https://developer.riotgames.com/apis#account-v1/GET_getByRiotId Riot Developer Portal developer.riotgames.com gameName => 닉네임tagLine => 태그 이름 한국은 아시아니까 아시아로 선택해주고미리 만들어준 키를 선택해준다 200이 뜨면 성공한거다 response body 이렇게 뜬다 여기서 알수있는데 puuid값이 나오는데 이걸로 매치 아이디를 통해서 전적검색이 가능해진다 그다음에https://developer.riotgames.com/apis#match-v5 Riot Developer Portal developer.riotgames.com이쪽으로 들어가준다 이걸 클릭해주고 아까..
깃액션에서 ci 할떄 나오는 문제이다 Error: Error: Dependency submission failed for dependency-graph-reports/java_ci_with_gra 뜰떄 있는https://docs.github.com/en/code-security/supply-chain-security/understanding-your-software-supply-chain/configuring-the-dependency-graph 이걸로 해결하면 좋을거같습니다.
1번문제 import java.util.*; public class Main { public static void main(String[] args) { Scanner scan = new Scanner(System.in); int n = scan.nextInt();// 괄호의 수 for (int i = 0; i < n; i++) { Stack stack = new Stack(); // 캐릭터타입 String s = scan.next(); for (int j = 0; j < s.length(); j++) { char currentChar = s.charAt(j); if (currentChar == '(' || currentChar == '{' || currentChar == '[') { stack.push..
1번문제 #include int sum(int num); int main(void) { int num; scanf_s("%d", &num); printf("%d", sum(num)); return 0; } int sum(int num) { if (num == 0) return 0; else if (num > 0) return num + sum(num - 1); } 2번문제 #include using namespace std; int pow(int a, int n) { if (n == 0) return 1; else return a * pow(a, n - 1); } int main() { int a, n; cout > a; cout > n; cout
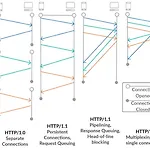
1.HTTP 이란 (HyperText Transfer Protocol) 웹 어플리 케이션 계층 프로토콜 (TCP 기반으로 동작) 웹 클라이언트가 웹페이지를 어떻게 요청(Response)하는지를 정의 Stateless Protocol RFC 1945 ,RFC 7230, RFC 7540에 정의 웹페이지 Hyper Text = Text +Link 객체들로 구성 2. HTTP 개요 Request- Response 구조 Client-Server 구조 URL(Uniform Resurce Locator) 네트 워크 상에 존재하는 자원의 위치를 명세 하기 위한 규약 HTTP는 TCP를 사용 데이터의 손실 혹은 순서는 HTTP가 고려하지 않으며, TCP가 해결 비연결성(Connectionless) 클라이언트와 서버가 연..
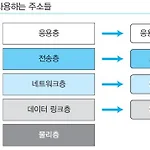
3개의 계층에서 사용되는 주소 물리 주소 논리 주소 포트 주소 응용 -특수 주소 물리 주소 링크 주소 WAN이나 LAN에서 정의된 노드의 주소 이더넷 네트워크 인터페이스 카드 (NIC) 의 6바이트 주소 근거리 통신망에서는 2바이트 마다 콜론(:)으로 나누어지는 16진수 12자리로 구성된 48비트(바이트) 논리 주소 인터넷 주소, IP주소 현재 인터넷에 연결된 호스트 식별 IPv4 32비트 주소 체계 2^32 또는 4294967296 (40억이상) IPv6 IPv4의 주소 고갈문제 를 해결하기 위해 대두 기존 IPv4의 32비트 주소체계를 128 비트로 확정 포트주소 컴퓨터는 다수의 프로세스를 동시에 실행함 한프로세스가 다른 프로세스와 통신을 함 프로세스를 식별하는 주소(16bit) 64*1024 개의 ..